The bar spacing and bar width are calculated depending on the number of bars (n) passed to the barchart() display function.
There is currently no vertical scale but this could be added...
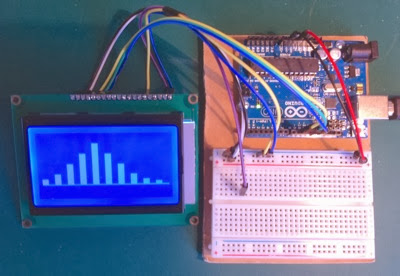
Code
#include "U8glib.h" // SPI SCK-EN, MOSI-RW & SS-CS #define EN 13 #define RW 11 #define CS 10 // display set up, bar, line position L & R #define BARWDTH 5 #define LINEY 50 #define LINEXL 4 #define LINEXR 124 #define BARS 10 U8GLIB_ST7920_128X64_1X lcd(EN, RW, CS); // serial use, PSB = GND void setup() { lcd.begin(); // init display lcd.setRot180(); // flip screen } void loop() { int barht[BARS] = {5, 10, 20, 30, 40, 30, 20, 10, 5, 3}; // ht of the BARS bars // ... other code barchart(BARS, barht); // draw HT bars display } // plot line and bar at position and height void barchart(int n, int bh[]) { int i, s, w; // bars, spacing and width s = (LINEXR - LINEXL) / n; w = s / 2; lcd.firstPage(); do { lcd.drawLine(LINEXL, LINEY, LINEXR, LINEY); for(i = 0; i < n; i++) { lcd.drawBox(LINEXL + s * i, LINEY - bh[i], w, bh[i]); } }while(lcd.nextPage()); }
I just discovered that the lcd.drawBox(...) function gets mighty confused if the last term is 0 (zero). So any implementation should make sure that is is at least 1.
No comments:
Post a Comment